Bungee jumping into Quarkus
Blindfolded but happy
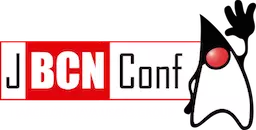
María Arias de Reyna Domínguez
@delawen@floss.social
Senior Software Engineer
María Arias de Reyna Domínguez
@delawen@floss.social
Senior Software Engineer
GraalVM
https://openjdk.org/projects/leyden/
Error: Substitution target for io.quarkus.runtime.generated.Target_org_jboss_logmanager_Logger is not loaded. Use field `onlyWith` in the `TargetClass` annotation to make substitution only active when needed.
com.oracle.svm.core.util.UserError$UserException: Substitution target for io.quarkus.runtime.generated. Target_org_jboss_logmanager_Logger is not loaded. Use field `onlyWith` in the `TargetClass` annotation to make substitution only active when needed.
Don't be me.
native
native
native
feb 02, 2022 11:22:19 A. M. io.quarkus.runtime.ApplicationLifecycleManager run ERROR: Failed to start application (with profile prod) java.lang.ClassNotFoundException: org.objectweb.asm.ClassVisitor at java.base/jdk.internal.loader.BuiltinClassLoader.loadClass(BuiltinClassLoader.java:641) at java.base/jdk.internal.loader.ClassLoaders$AppClassLoader.loadClass(ClassLoaders.java:188) at java.base/java.lang.ClassLoader.loadClass(ClassLoader.java:520) at io.smallrye.config.ConfigMappingInterface.getClassBytes(ConfigMappingInterface.java:152) at io.smallrye.config.ConfigMappingLoader.loadClass(ConfigMappingLoader.java:98) at io.smallrye.config.ConfigMappingLoader.getImplementationClass(ConfigMappingLoader.java:81) at io.smallrye.config.ConfigMappingLoader$1.computeValue(ConfigMappingLoader.java:20) at io.smallrye.config.ConfigMappingLoader$1.computeValue(ConfigMappingLoader.java:17) at java.base/java.lang.ClassValue.getFromHashMap(ClassValue.java:228) at java.base/java.lang.ClassValue.getFromBackup(ClassValue.java:210) at java.base/java.lang.ClassValue.get(ClassValue.java:116) at io.smallrye.config.ConfigMappingLoader.configMappingObject(ConfigMappingLoader.java:57) at io.smallrye.config.ConfigMappingContext.constructGroup(ConfigMappingContext.java:80) at io.kaoto.backend.api.metadata.catalog.ViewDefinitionRepository1270545574Impl.<init>(Unknown Source)
org.jboss.jandex
jandex-maven-plugin
1.2.1
make-index
jandex
@ApplicationScoped
public class ClusterService {
@Inject
MyOtherBean beanybeany;
@Counted
String translate(String sentence) {
System.out.println("Interceptor binding annotation from MicroProfile");
}
@PostConstruct
void init() {
System.out.println("Hello there!");
}
@PreDestroy
void init() {
System.out.println("My revenge will be terrible.");
}
}
@Startup
@ApplicationScoped
public class StepCatalog extends AbstractCatalog {
private Instance<StepCatalogParser> stepCatalogParsers;
@Inject
public void setStepCatalogParsers(
final Instance<StepCatalogParser> stepCatalogParsers) {
this.stepCatalogParsers = stepCatalogParsers;
}
@Inject
void multipleBeans(OneBean one, AnotherBean another) {
this.one = one;
this.another = another;
}
}
@RegisterForReflection
public class MyObjectDeserializer implements JsonbDeserializer {
private static final Jsonb JSONB = JsonbBuilder.create();
@Override
public MyObject deserialize(final JsonParser parser,
final DeserializationContext context,
final Type rtType) {
String type = parser.getObject().toString().getString("type");
switch (type) {
case "patata":
...
}
}
@JsonSubTypes({
@JsonSubTypes.Type(value = Patata.class, name = "patata"),
@JsonSubTypes.Type(value = Poteito.class, name = "potato")})
//This is a workaround utility class until Quarkus supports fully polymorphism
@JsonbTypeDeserializer(MyObjectDeserializer.class)
public abstract class MyObject implements Cloneable {
...
}
public CompletableFuture<List<T>> doIt() {
CompletableFuture<List<T>> res = new CompletableFuture<>();
res.completeAsync(() -> doItSlowly());
return res;
}
public void doItAndwait() {
doIt().join();
}
WARNING [io.ver.cor.imp.BlockedThreadChecker] (vertx-blocked-thread-checker) Thread Thread[vert.x-eventloop-thread-10,5,main]=Thread[vert.x-eventloop-thread-10,5,main] has been blocked for 4827 ms, time limit is 2000 ms: io.vertx.core.VertxException: Thread blocked
@NoCache
@Path("/{name}/logs")
@Produces(MediaType.TEXT_PLAIN)
@GET
@Operation(summary = "Get logs",
description = "Get the resource's log.")
@Blocking
public Multi<String> logs(
final @Parameter(description = "Name of the resource "
+ "of which logs should be retrieved.")
@PathParam("name") String name,
final @Parameter(description = "Namespace of the cluster "
+ "where the resource is running.")
@QueryParam("namespace") String namespace,
final @Parameter(description = "Number of last N lines to be "
+ "retrieved.")
@QueryParam("lines") int lines) {
return clusterService.streamlogs(namespace, name, lines);
}
@QuarkusTest
@TestHTTPEndpoint(IntegrationResource.class)
class IntegrationResourceTest {
@Test
void thereAndBackAgain() throws URISyntaxException, IOException {
var res = given()
.when()
.contentType("application/json")
.body(Collections.emptyList())
.post("/dsls")
.then()
.statusCode(Response.Status.OK.getStatusCode());
List<String> dsls =
mapper.readValue(res.extract().body().asString(), List.class);
assertFalse(dsls.isEmpty());
}
}
@WithKubernetesTestServer
@QuarkusTest
class ClusterServiceTest {
@Inject
public void setKubernetesClient(final KubernetesClient kubernetesClient) {
this.kubernetesClient = kubernetesClient;
}
private KubernetesClient kubernetesClient;
}
quarkus-universe-bom
io.quarkus
2.10.2.Final
${quarkus.platform.version}